khan Waseem
Fri Jan 27 2023
-5 min read
What is Design Pattern?
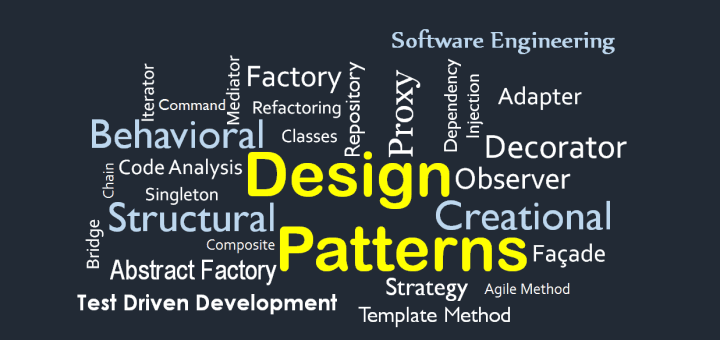
Design Patterns: Building Blocks of Maintainable Software
Design patterns are recurring solutions to common problems encountered in software design and development. They are like templates that provide a blueprint for solving particular issues while building software systems. Design patterns are essential because they encapsulate best practices, making it easier for developers to create maintainable and reusable code.
The Need for Design Patterns
In the realm of software development, numerous challenges are encountered repeatedly. These challenges include creating objects, managing dependencies, extending functionality, enabling object communication, handling errors, and ensuring concurrency and multithreading. Design patterns address these issues by offering well-established solutions.
Object Creation: Design patterns help in creating objects efficiently, ensuring they are constructed correctly and promoting reusability.
Managing Dependencies: They guide developers in managing dependencies between classes and components, reducing coupling and facilitating changes.
Extending Functionality: Design patterns allow developers to add new features or modify existing ones without disrupting the existing codebase.
Object Communication: They provide guidelines for enabling objects to communicate and collaborate without creating tight and brittle connections between them.
Error Handling: Design patterns assist in handling errors and exceptions consistently and gracefully throughout the application.
Concurrency and Multithreading: They offer solutions for designing software that functions correctly and efficiently in concurrent and multithreaded environments.
Understanding Design Patterns
Design patterns are not code snippets to be copied and pasted into a project. Instead, they are high-level descriptions of solutions to recurring design problems. A design pattern typically includes:
Pattern Name: A descriptive name for the pattern (e.g., Singleton or Observer).
Problem: An explanation of the specific design problem the pattern addresses.
Solution: Details on how the pattern solves the problem, including the structure of classes and objects involved, their relationships, and their responsibilities.
Consequences: A discussion of the trade-offs and considerations when using the pattern, such as its impact on flexibility, extensibility, and performance.
Examples: Real-world code examples in various programming languages to illustrate pattern implementation.
Categories of Design Patterns
Design patterns are categorized into three main groups:
1. Creational Patterns
Creational patterns deal with object creation, abstracting it to make a system independent of how objects are created, composed, and represented. Common creational patterns include:
Singleton Pattern: Ensures a class has only one instance and provides global access to it.
Factory Method Pattern: Defines an interface for creating objects but lets subclasses alter the type of objects that will be created.
Abstract Factory Pattern: Provides an interface for creating families of related or dependent objects without specifying their concrete classes.
Builder Pattern: Separates the construction of a complex object from its representation, allowing the same construction process to create different representations.
Prototype Pattern: Creates new objects by copying an existing object, known as the prototype.
2. Structural Patterns
Structural patterns focus on class and object composition, defining relationships between objects to form larger structures. They include:
Adapter Pattern: Allows the interface of an existing class to be used as another interface.
Bridge Pattern: Separates an object’s abstraction from its implementation, allowing them to vary independently.
Composite Pattern: Composes objects into tree structures to represent part-whole hierarchies, treating individual objects and compositions uniformly.
Decorator Pattern: Attaches additional responsibilities to objects dynamically, offering a flexible alternative to sub classing.
Facade Pattern: Provides a simplified interface to a complex subsystem, making it easier to use.
Flyweight Pattern: Minimizes memory usage or computational expenses by sharing as much as possible with related objects.
3. Behavioral Patterns
Behavioral patterns address how objects interact and communicate with one another. They define communication patterns to promote flexibility and reusability. Key behavioral patterns include:
Chain of Responsibility Pattern: Passes a request along a chain of handlers, with each handler deciding either to process the request or pass it to the next handler.
Command Pattern: Encapsulates a request as an object, enabling parameterization of clients with requests and operations.
Interpreter Pattern: Evaluates language grammar or expressions, defining a grammar and an interpreter to interpret it.
Iterator Pattern: Provides a way to access elements of an aggregate object sequentially without exposing its underlying representation.
Mediator Pattern: Defines an object that centralizes communication between other objects, reducing dependencies between them.
Memento Pattern: Captures and externalizes an object’s internal state for later restoration.
Observer Pattern: Establishes a one-to-many dependency between objects, so when one changes state, its dependents are notified and updated automatically.
State Pattern: Allows an object to alter its behavior when its internal state changes, appearing to change its class.
Strategy Pattern: Defines a family of algorithms, encapsulating each one and making them interchangeable, independently from clients.
Template Method Pattern: Defines the skeleton of an algorithm in the superclass but lets subclasses override specific steps without changing the structure.
Visitor Pattern: Represents an operation to be performed on elements of an object structure, enabling the definition of new operations without changing element classes.
Benefits of Using Design Patterns
The adoption of design patterns offers several significant advantages in software development:
Proven Solutions: Design patterns encapsulate best practices, offering proven solutions to common problems that have been refined by experienced developers over time.
Code Reusability: Patterns promote code reuse, allowing developers to apply established solutions instead of reinventing the wheel, saving time and effort.
Improved Code Maintainability: Design patterns lead to more modular and organized code, making it easier to understand, modify, and maintain software systems, even as they grow in complexity.
Enhanced Collaboration: Patterns provide a common vocabulary and structure for discussing and sharing design ideas, facilitating collaboration among developers.
Scalability: Software designed with patterns can accommodate future changes and feature additions, making it more scalable and adaptable.
Flexibility: Patterns encourage loose coupling and encapsulation, making it easier to swap components or change system behavior.
Considerations When Using Design Patterns
While design patterns offer numerous benefits, it’s essential to use them judiciously:
Problem Relevance: Not all problems require design patterns. Applying patterns unnecessarily can lead to over-engineered and complex solutions.
Development Context: The choice of pattern should consider the specific project requirements and constraints, as patterns may vary in suitability.
Maintenance: Patterns should be maintained along with the codebase to ensure they remain effective as the software evolves.
Understanding: Developers must thoroughly understand the patterns they use to apply them correctly.
In conclusion, design patterns are fundamental building blocks of maintainable and reusable software. They provide tested and proven solutions to common development challenges, improving code quality and fostering collaboration among developers. While patterns offer significant benefits, they should be applied thoughtfully, considering the problem at hand and the context of the development project.