khan Waseem
Fri Jan 27 2023
-4 min read
What is Express? Building Web Applications with Node.js
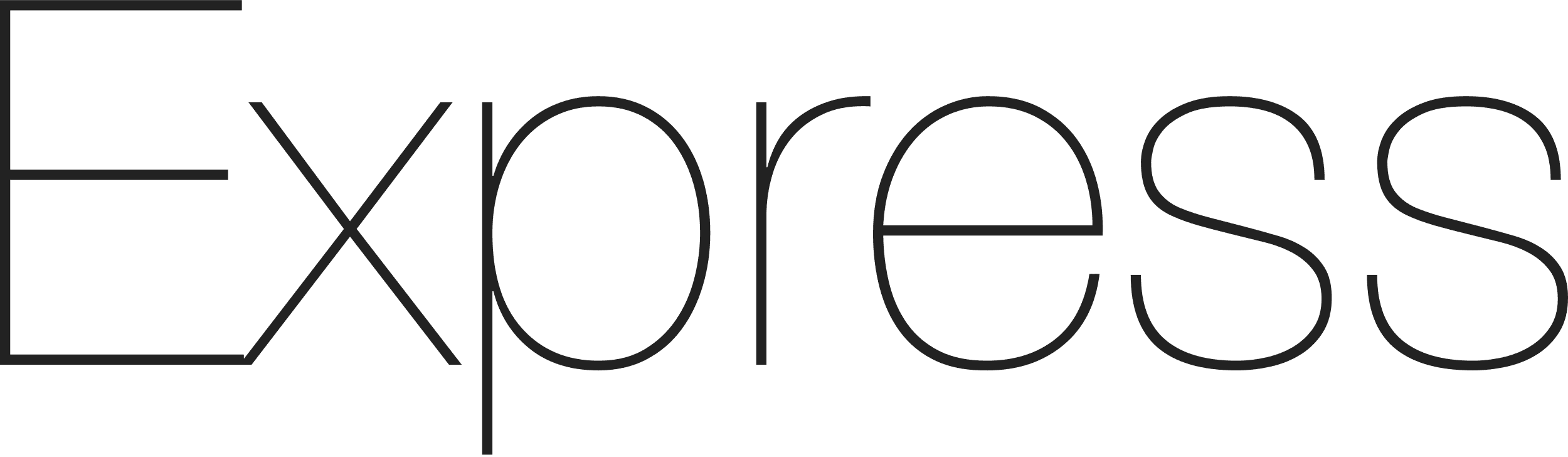
Express.js, often referred to as Express, is a popular and minimalist web application framework for Node.js. It is designed for building robust and scalable web applications and APIs quickly and easily. In this comprehensive guide, we will explore what Express is, its core features, and provide multiple examples to illustrate its capabilities.
Introduction to Express.js
Express.js, commonly known as Express, is a web application framework for Node.js, a runtime environment for executing JavaScript on the server-side. It was developed by TJ Holowaychuk and is now maintained by the Node.js Foundation. Express.js is renowned for its simplicity and flexibility, making it an ideal choice for building web applications and APIs.
Key Features of Express.js
Express.js provides a wide range of features and functionalities that simplify web application development:
1. Routing:
- Express simplifies route definition and management, making it easy to handle HTTP requests.
- Routes can be defined for various HTTP methods (GET, POST, PUT, DELETE, etc.) and URL patterns.
2. Middleware:
- Middleware functions can be used to process requests and responses in a modular and reusable way.
- Middleware can be applied globally or to specific routes, allowing for fine-grained control over request handling.
3. Templating Engines:
- Express supports various templating engines like EJS, Pug (formerly Jade), and Handlebars.
- These engines make it convenient to generate dynamic HTML pages on the server.
4. Static Files:
- Serving static files, such as CSS, JavaScript, and images, is straightforward with Express.
- The
express.static
middleware serves static files from a specified directory.
5. Error Handling:
- Express provides a built-in error-handling mechanism using middleware.
- Custom error handling can be defined to gracefully handle application errors.
6. Request and Response Handling:
- Express simplifies request and response handling with features like query parameters, request bodies, and response status codes.
- Middleware can be used to validate, sanitize, or modify incoming requests and outgoing responses.
7. Integration with Databases:
- Express can be integrated with various databases, including relational databases (e.g., MySQL, PostgreSQL) and NoSQL databases (e.g., MongoDB).
- This flexibility allows you to choose the database that best suits your application’s needs.
8. Session and Authentication:
- Sessions and authentication can be implemented using middleware or third-party packages.
- Popular authentication packages like Passport.js can be easily integrated with Express.
9. REST API Development:
- Express is widely used for building RESTful APIs due to its simplicity and performance.
- It provides an ideal platform for creating APIs for mobile apps, web applications, and other clients.
10. WebSocket Support:
- While Express is primarily designed for HTTP, it can be combined with WebSocket libraries like Socket.io for real-time communication.
Code language: SQL (Structured Query Language) (sql)
Express.js Example: Creating a RESTful API
To demonstrate Express.js in action, we will build a simple RESTful API for managing tasks. This example will showcase key Express features, including routing, middleware, and request handling.
Step 1: Project Setup
First, ensure you have Node.js and npm (Node Package Manager) installed on your system. Then, create a new directory for your Express project and initialize it with npm:
mkdir express-api-example
cd express-api-example
npm init -y
Code language: Bash (bash)
Next, install Express as a project dependency:
npm install express
Code language: Bash (bash)
Step 2: Creating the Express Application
Create an app.js
file in your project directory and set up your Express application:
const express = require('express');
const app = express();
const port = 3000;
app.use(express.json());
// Define a simple array to store tasks
const tasks = [];
// Define routes for managing tasks
app.get('/tasks', (req, res) => {
res.json(tasks);
});
app.post('/tasks', (req, res) => {
const task = req.body;
tasks.push(task);
res.status(201).json(task);
});
app.get('/tasks/:id', (req, res) => {
const id = parseInt(req.params.id);
const task = tasks.find((t) => t.id === id);
if (!task) {
res.status(404).json({ message: 'Task not found' });
} else {
res.json(task);
}
});
app.put('/tasks/:id', (req, res) => {
const id = parseInt(req.params.id);
const updatedTask = req.body;
const index = tasks.findIndex((t) => t.id === id);
if (index === -1) {
res.status(404).json({ message: 'Task not found' });
} else {
tasks[index] = updatedTask;
res.json(updatedTask);
}
});
app.delete('/tasks/:id', (req, res) => {
const id = parseInt(req.params.id);
const index = tasks.findIndex((t) => t.id === id);
if (index === -1) {
res.status(404).json({ message: 'Task not found' });
} else {
tasks.splice(index, 1);
res.json({ message: 'Task deleted' });
}
});
app.listen(port, () => {
console.log(`Express server is running on port ${port}`);
});
Code language: JavaScript (javascript)
In this code:
- We import the Express module, create an instance of the Express application, and define the port number.
- We use
express.json()
middleware to parse JSON request bodies. - We define an array called
tasks
to store task objects. - We create routes for handling tasks, including listing tasks, creating tasks, retrieving a specific task, updating a task, and deleting a task.
- The server listens on port 3000.
Step 3: Running the Express Application
You can start the Express application by running the following command in your project directory:
node app.js
Code language: Bash (bash)
The server will start, and you can access the API at http://localhost:3000/tasks
.
Here are some example requests you can make to interact with the API:
- GET /tasks: Retrieve a list of tasks.
- POST /tasks: Create a new task.
- GET /tasks/{id}: Retrieve a specific task by ID.
- PUT /tasks/{id}: Update a specific task by ID.
- DELETE /tasks/{id}: Delete a specific task by ID.
This example showcases how Express.js simplifies the creation of a RESTful API, from routing and request handling to error management and response generation.
Conclusion
Express.js is a powerful and flexible framework for building web applications and APIs with Node.js. Its simplicity and extensive ecosystem of middleware and packages make it a top choice for developers when creating server-side applications. Whether you’re building a small web application or a complex API, Express.js provides the tools and flexibility needed to get the job done efficiently and effectively. As you delve deeper into web development with Node.js, mastering Express.js will be a valuable skill that allows you to build robust and scalable applications.