khan Waseem
Thu Jan 26 2023
-3 min read
What is python?
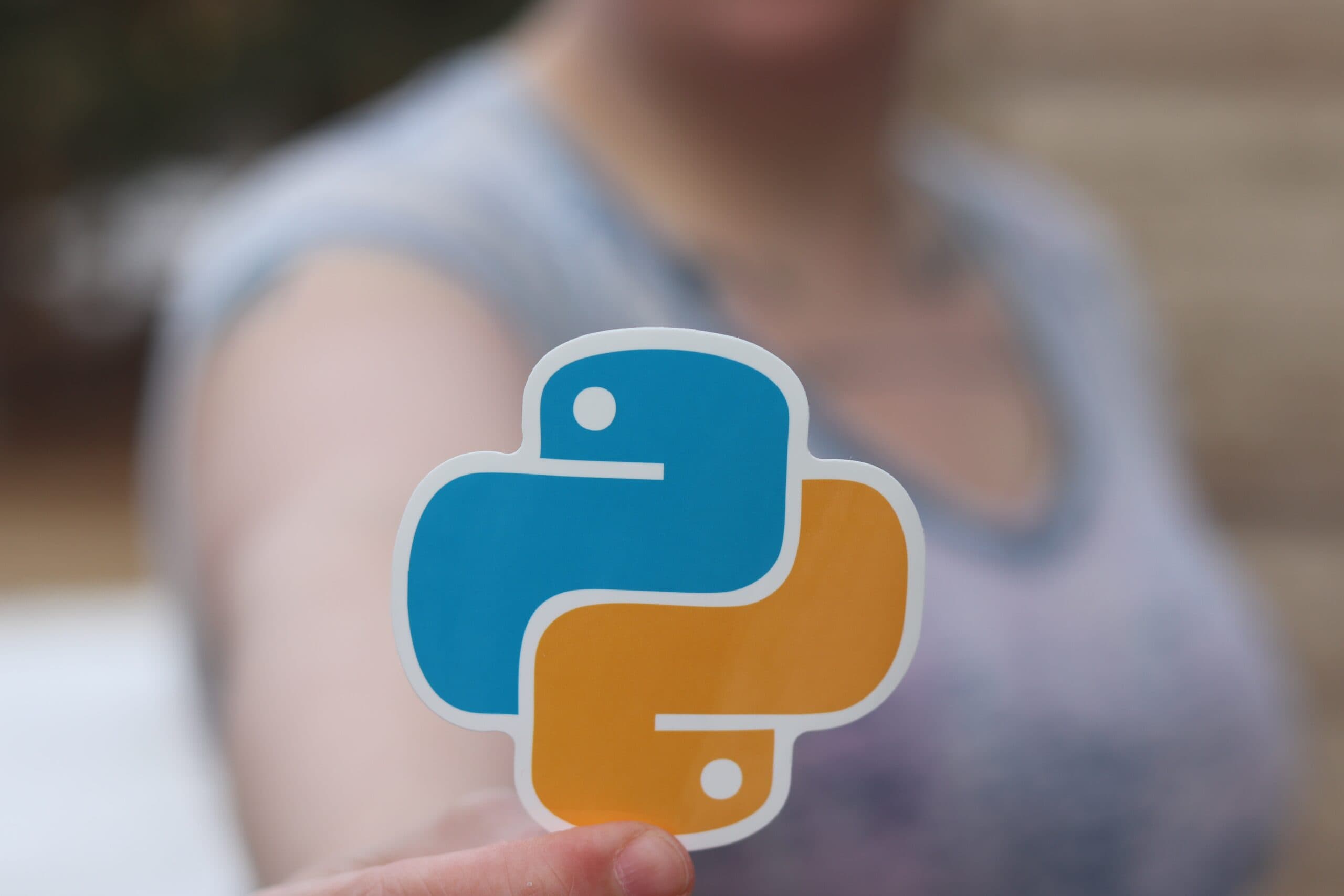
Python is a high-level, interpreted programming language that is widely used for a variety of purposes, including web development, data science, artificial intelligence, and scientific computing. It was first released in 1991 by Guido van Rossum and has since become one of the most popular programming languages in the world.
One of the most notable features of Python is its simplicity and readability. The language has a relatively clean and straightforward syntax, which makes it easy for beginners to learn and for experienced developers to read and understand. Python also supports a wide range of programming paradigms, including object-oriented, imperative, and functional programming.
Python has a simple and easy-to-learn syntax, which makes it a popular choice for beginners and experienced developers alike. The language also has a wide range of libraries and frameworks, which makes it easy to perform a variety of tasks, from web development to data science.
Python is often used for web development, thanks to its wide range of web frameworks such as Django and Flask. These frameworks make it easy to build and deploy web applications, and they provide many built-in features such as database integration, user authentication, and form handling.
Data science and machine learning are other popular areas where Python is used. Python has a vast number of libraries such as NumPy, pandas, and scikit-learn that are used for data manipulation and analysis, as well as libraries like TensorFlow and Keras that are used for machine learning and deep learning.
Python is also used in scientific computing, thanks to its powerful libraries such as NumPy, SciPy, and Matplotlib. These libraries provide powerful tools for working with large datasets, linear algebra, and complex mathematical functions.
Python also has a large and active community, which has resulted in the development of a wide range of libraries and frameworks for various purposes. This makes it easy for developers to find pre-existing libraries and modules to use in their projects, which can save a lot of time and effort.
Here is a simple example of a Python program that will print “Hello, World!” to the console:
print("Hello, World!")
Code language: Python (python)
This program uses the built-in print()
function to output the string “Hello, World!” to the console. The print()
function is a common way to output data in Python and is used in many programs.
Python can also be used to perform mathematical operations. Here is an example that calculates the area of a circle with a radius of 5:
import math
radius = 5
area = math.pi * (radius ** 2)
print(area)
Code language: Python (python)
In this example, the math
library is imported at the top of the program. This library contains many mathematical functions, including pi
, which is used to calculate the area of the circle. The variable radius
is set to 5 and the area is calculated using the formula math.pi * (radius ** 2)
. The result is then printed to the console using the print()
function.
Python is commonly used in web development, thanks to its wide range of web frameworks such as Django and Flask. These frameworks make it easy to build and deploy web applications, and they provide many built-in features such as database integration, user authentication, and form handling. Here is an example of a simple web server using the Flask framework:
from flask import Flask
app = Flask(__name__)
@app.route("/")
def hello():
return "Hello, World!"
if __name__ == "__main__":
app.run()
Code language: Python (python)
Data science and machine learning are other popular areas where Python is used. Python has a vast number of libraries such as NumPy, pandas, and scikit-learn that are used for data manipulation and analysis, as well as libraries like TensorFlow and Keras that are used for machine learning and deep learning.
For example, using the pandas library, we can read a CSV file, display the first five rows, and calculate the mean of a column:
import pandas as pd
data = pd.read_csv("data.csv")
print(data.head())
print(data["column_name"].mean())
Code language: Python (python)
Python is also used in scientific computing, thanks to its powerful libraries such as NumPy, SciPy, and Matplotlib.